Sup guys! I hope everyone is doing well. In this blog, i will be sharing with you about my learning experience on ARDUINO PROGRAMMING, something that is interesting to study and play around with. In fact, this is the first time I get to learn how to code and how to modify the codes to achieve our objective. As a chemical engineer in SP, it came as no surprise that my team accomplished all the challenges as required by our teacher. With SP, it's SO POSSIBLE :)
There are 4 tasks that will be explained in this page: 1.Input devices:
a.Interface a potentiometer analog input to maker UNO board and
measure/show its signal in serial monitor Arduino IDE.
b.Interface a LDR to maker UNO board and measure/show its signal in
serial monitor Arduino IDE
2.Output devices:
a.Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc)
b.Include the pushbutton on the MakerUno board to start/stop part 2.a. above
For each of the tasks, I will describe:
1.The program/code that I have used and explanation of the code. The code is in writable format (not an image).
2.The sources/references that I used to write the code/program.
3.The problems I encountered and how I fixed them.
4.The evidence that the code/program worked in the form of video of the executed program/code.
Finally, I will describe:
5.My Learning reflection on the overall Arduino programming activities.
Input devices: Interface a potentiometer analog input to maker UNO board and measure/show its signal in serial monitor Arduino IDE. 1.Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int sensorPin = A0; int ledPin = 13; int sensorValue = 0; void setup() { pinMode(ledPin,OUTPUT); } void loop() { sensorValue = analogRead(sensorPin); digitalWrite(ledPin, HIGH); delay(sensorValue); digitalWrite(ledPin, LOW); delay(sensorValue); } | SensorPin is set as A0 LEDPIN is set at 13 Sensor Value is set as 0 Within the void setup: LEDPIN set PIN 13 as the OUTPUT Inside the void loop: sensorValue = analogRead(sensorPin) reads the value of sensorPin, in this case it is set A0 at the start and write it into an integer variable. digitalWrite(ledPin, HIGH) Turns on LedPin (L13) digitalWrite(ledPin, LOW) Turns off LedPin (L13) delay(sensorValue) The value of delay time changes with the sensor value |
2.Below are the hyperlink to the sources/references that I used to write the code/program.
Hyperlink
3.Below are the problems I have encountered and how I fixed them.
I believe that I only encountered a few problems with the connection of the wires when trying to connect the potential meter to the breadboard. I fixed the problem by taking out the wires and re-install them, fortunately enough it only took me one try to make my program work.
4.Below is the short video as the evidence that the code/program work.
Input devices: Interface a LDR to maker UNO board and measure/show its signal in serial monitor Arduino IDE:
1.Below are the code/program I have used and the explanation of the code
Code/program in writeable format | Explanation of the code |
int LDR = A0; int ledPin = 13; int LDRvalue = 0; void setup() { pinMode(ledPin,OUTPUT); } void loop() { LDRvalue = analogRead(LDR); if(LDRvalue > 600) digitalWrite(ledPin, HIGH); else digitalWrite(ledPin, LOW); } | LDR is set as A0 LEDPIN is set as 13 LDRvalue is set to be 0 Inside the void setup: pinMode(ledPin,OUTPUT) LEDPIN 13 was set as output Inside the void loop: LDRvalue = analogRead(LDR) Reads the value of LDR (A0) and write it as an integer variable. if(LDRvalue > 600)digitalWrite(ledPin, HIGH) Turns on the LEDPIN (L13) if LDRvalue is more than 600. else digitalWrite(ledPin, LOW) Otherwise turns off the LEDPIN (L13) |
2.Below are the hyperlink to the sources/references that I used to write the code/program.
3.Below are the problems I have encountered and how I fixed them.
I have encountered several problems during the process.
First of all, I did not aligh the resistor and the LDR correctly and as a result, my LDR was not light up.
Secondly, I neglected there was supposed to be a wire before the LDR that is connected on breadboard. I managed to fix the two problems mentioned by revisiting the learning package on brightspace and compared the correct design to my version.
Lastly,I tried to use my hand to cover the LDR when the circuit was successfully built, however, LED at L13 was not light up. I quickly fixed this problem by using my cloth to cover the LDR to fulfill the sensor requirement for the LED to light up.
4.Below is the short video as the evidence that the code/program work.
Output devices: Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc)
1.Below are the code/program I have used and the explanation of the code
Code/program in writeable format | Explanation of the code |
const int greenLedVehicle = 5; const int yellowLedVehicle = 6; const int redLedVehicle = 7; const int greenLedPedestrian = 3; const int redLedPedestrian = 4; void setup() { pinMode(greenLedVehicle, OUTPUT); pinMode(yellowLedVehicle, OUTPUT); pinMode(redLedVehicle, OUTPUT); pinMode(greenLedPedestrian, OUTPUT); pinMode(redLedPedestrian, OUTPUT); digitalWrite(greenLedVehicle, HIGH); digitalWrite(redLedPedestrian, HIGH); } void loop() { digitalWrite(greenLedVehicle, LOW); digitalWrite(yellowLedVehicle, HIGH); delay(2000); digitalWrite(yellowLedVehicle, LOW); digitalWrite(redLedVehicle, HIGH); delay(1000); digitalWrite(redLedPedestrian, LOW); digitalWrite(greenLedPedestrian, HIGH); delay(5000); digitalWrite(greenLedPedestrian, LOW); digitalWrite(redLedPedestrian, HIGH); delay(1000); digitalWrite(redLedVehicle, LOW); digitalWrite(greenLedVehicle, HIGH); delay(2000); } | GreenLedVehicle is set to be PIN 5 YellowLedVehicle is set to be PIN 6 RedLedVehicle is set to be PIN 7 GreenLedPedstrain is set to be PIN 3 RedLedPedstrain is set to be PIN 4 Inside the void setup: pinMode(greenLedVehicle, OUTPUT);pinMode(yellowLedVehicle, OUTPUT);pinMode(redLedVehicle, OUTPUT);pinMode(greenLedPedestrian, OUTPUT);pinMode(redLedPedestrian, OUTPUT) PIN 3,4,5,6,7 are set as OUTPUT digitalWrite(greenLedVehicle, HIGH); PIN 5 will be on at the start digitalWrite(redLedPedestrian, HIGH) PIN 4 will be on at the start Inside the void loop: digitalWrite(greenLedVehicle, LOW);digitalWrite(yellowLedVehicle, HIGH);delay(2000); PIN 5 will be turned off first, followed by turning on PIN 6. There will be a 2 seconds delay time in between the actions. digitalWrite(yellowLedVehicle, LOW);digitalWrite(redLedVehicle, HIGH);delay(1000); PIN 6 will be turned off first, followed by turning on PIN 7. There will be a 1 second delay time in between the actions. digitalWrite(redLedPedestrian, LOW);digitalWrite(greenLedPedestrian, HIGH);delay(5000); PIN 7 will be turned off first, followed by turning on PIN 5. There will be a 5 seconds delay time in between the actions. digitalWrite(greenLedPedestrian, LOW);digitalWrite(redLedPedestrian, HIGH);delay(1000); digitalWrite(redLedVehicle, LOW);digitalWrite(greenLedVehicle, HIGH);delay(2000); PIN 3 will be turned off first, followed by turning on PIN 2. Delay time will be 1 second. PIN 2 will be turned off, followed by turning on PIN 3. Delay will be 2 seconds. |
2.Below are the hyperlink to the sources/references that I used to write the code/program.
Hyperlink
3.Below are the problems I have encountered and how I fixed them.
At first, the code that I designed only allowed the "traffic light" to start changing colour when I pressed the pushbotton. Moreover, the cycle would not repeat by itself, should the pushbutton was not pressed.
After deliberating the reason why it did not work out and discussing with my teammate Aminur, we realised that there were some flaws in the code we copied and pasted from Brightspace. Kudos to Aminur, he spotted the mistakes and we were able to modify the code to make it work. BRAVOOOOOOOOOOOO!
4.Below is the short video as the evidence that the code/program work.
Output devices: Include pushbutton to start/stop the previous task
1.Below are the code/program I have used and the explanation of the code
Code/program in writeable format | Explanation of the code |
const int greenLedVehicle = 5; const int yellowLedVehicle = 6; const int redLedVehicle = 7; const int greenLedPedestrian = 3; const int redLedPedestrian = 4; const int pushButton = 2; void setup() { pinMode(greenLedVehicle, OUTPUT); pinMode(yellowLedVehicle, OUTPUT); pinMode(redLedVehicle, OUTPUT); pinMode(greenLedPedestrian, OUTPUT); pinMode(redLedPedestrian, OUTPUT); pinMode(pushButton, INPUT_PULLUP); digitalWrite(greenLedVehicle, HIGH); digitalWrite(redLedPedestrian, HIGH); } void loop() { if(digitalRead(pushButton) == LOW) { digitalWrite(greenLedVehicle, LOW); digitalWrite(yellowLedVehicle, HIGH); delay(2000); digitalWrite(yellowLedVehicle, LOW); digitalWrite(redLedVehicle, HIGH); delay(1000); digitalWrite(redLedPedestrian, LOW); digitalWrite(greenLedPedestrian, HIGH); delay(5000); digitalWrite(greenLedPedestrian, LOW); digitalWrite(redLedPedestrian, HIGH); delay(1000); digitalWrite(redLedVehicle, LOW); digitalWrite(greenLedVehicle, HIGH); } } | GreenLedVehicle is set to be PIN 5 YellowLedVehicle is set to be PIN 6 RedLedVehicle is set to be PIN 7 GreenLedPedstrain is set to be PIN 3 RedLedPedstrain is set to be PIN 4 Pushbutton is set to be PIN 2 Insider the void setup: pinMode(greenLedVehicle, OUTPUT);pinMode(yellowLedVehicle, OUTPUT);pinMode(redLedVehicle, OUTPUT);pinMode(greenLedPedestrian, OUTPUT);pinMode(redLedPedestrian, OUTPUT); PIN 3,4,5,6,7 are set as OUTPUT pinMode(pushButton, INPUT_PULLUP) Pushbutton is set as INPUT digitalWrite(greenLedVehicle, HIGH);digitalWrite(redLedPedestrian, HIGH) PIN 4 and PIN 5 will be on at the start, before the pushbutton is pressed. Inside the void loop: if(digitalRead(pushButton) == LOW){digitalWrite(greenLedVehicle, LOW);digitalWrite(yellowLedVehicle, HIGH);delay(2000) If the pushbutton is pressed, PIN 5 will turn off while PIN 6 will be light up and there will be a delay of 2 seconds. digitalWrite(yellowLedVehicle, LOW);digitalWrite(redLedVehicle, HIGH);delay(1000); PIN 6 will be off while PIN 7 will be on. There will be a delay time of 1 second digitalWrite(redLedPedestrian,LOW); digitalWrite(greenLedPedestrian, HIGH);delay(5000); PIN 7 will be off while PIN 6 will be on.There is a delay time of 5 seconds. digitalWrite(greenLedPedestrian, LOW);digitalWrite(redLedPedestrian, HIGH);delay(1000) PIN 3 will be off while PIN 4 will be on. There is a delay time of 1 second. digitalWrite(redLedVehicle, LOW);digitalWrite(greenLedVehicle, HIGH) PIN 7 will be turned off and PIN 5 will be turned on.
|
2.Below are the hyperlink to the sources/references that I used to write the code/program.
Hyperlink
3.Below are the problems I have encountered and how I fixed them.
Frankly speaking, I did not encounter any problem completing this task as the code was available on Brightspace and all I needed to do was simply copy and paste the code onto my Arduino IDE, plus connecting the LEDs on breadboard and attaching the wires and resistors accordingly.
As the old saying goes, failure is the mother of success. After failing to attach the wire and resistor correctly, I learnt my lesson and got things working smoothly on my first attempt.
4.Below is the short video as the evidence that the code/program work.
Below is my Learning Reflection on the overall Arduino Programming activities.
It's always inspiring to look back at what we have achieved and what we can learn from our learning experience. Having completed all the tasks on Arduino Programming is no exception.
To begin with, it equipped me with some coding knowledge after studying the foundamentals of Arduino programme. As a chemical engineer, I did not expect myself learning this before the practical. However, after the completion of the lesson and practical, I am confident to say that I have acquired an useful skill and I personally believe that it will elevate myself to a new level in spite of the practical being somewhat superficial in terms of coding knowledge.
Now Let's jump into the activities/tasks I completed during the practical and the lesson. I learnt how to use a void setup and void loop to make our programme much more efficient instead of copy and paste it for many times. I also learnt that a resistor must be installed along with the LED to prevent it from the occurence of a short circuit, which will lead to the damage of LED. When perfoming the third and the fourth task, I learnt the importance of setting the delay time, as it will affect the time taken before the program proceeds to the next step.
For example, delay(1000) means the program will pause for 1 second (1000ms=1s) before taking the next step. A change from delay (1000) to delay (200) will make the program react much faster.
I had a practical on Arduino as well this week, which involved a lot of hands-on activities and coding work. Below is the picture of our gorgeous unicorn.
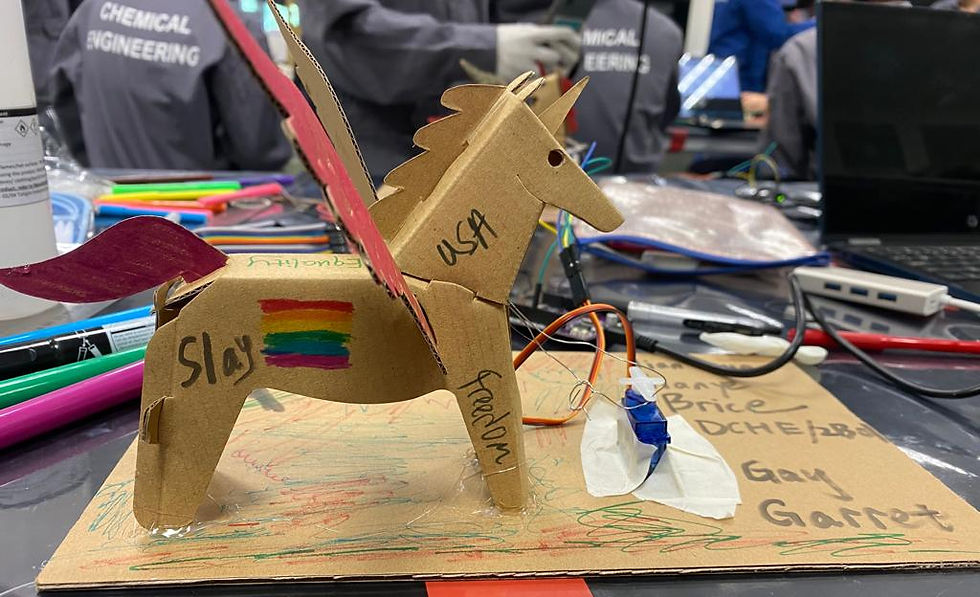
With the help of my teammates Jian Lun and Brice, we came up with our product "Gay Garret", which is able to flap naturally with great amplitude with the aid of servo and the servo will turn 5 times when the music is played. We achieved this by inserting the code of servo after the tonemelody part while keeping them in the same void loop as there can only be one and only void loop inside the Arduino Programe.
All in all, it was very fun to play around with Arduino programe. Since my group will be constructing a CO system that will vent out air with high CO concentration, we will be using two motors in the future prototyping process with the help of Arduino Programe. It is a great experience for both my group and myself as we will be better prepared for the future activities in CA2.
Comments